Secondary Marketplace
Introdution
The marketplace app allows token owners to list their NFTs for sale, make offers, and create auctions. Buyers can browse listings, fill offers, place bids, and execute transactions on-chain through smart contracts. The app enables a seamless off-chain experience for offer filling and bidding, with on-chain execution for security and transparency.
Deployments
Sepolia Testnet
Name of the Contract | Contract address |
---|---|
MarketplaceV1_1 | 0x2BAefd2ef0557d8E0fB9463d30651F59EE58d6c0 |
Architecture Overview
The architecture of a Secondary Marketplace typically consists of the following components:
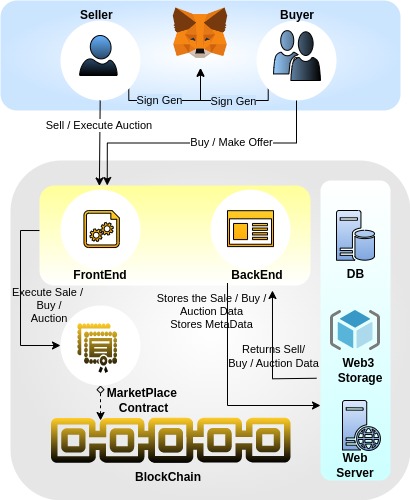
- Frontend Application: The frontend application serves as the user interface through which token owners and buyers interact with the marketplace. It provides screens for listing NFTs, making offers, participating in auctions, and facilitating off-chain interactions.
- Marketplace Backend: The backend of the marketplace app handles various functionalities such as processing listing requests, managing offers and auctions, and interacting with the blockchain.
- Centralized Database: The centralized database stores information related to NFT listings, offers, auction details, user accounts, and other relevant data. It is used to maintain the state of the marketplace and provide quick access to information.
- Smart Contracts: Smart contracts are deployed on the blockchain platform (e.g., Ethereum) and handle the on-chain execution of offers and auctions. They contain the logic for verifying transactions, transferring ownership, and maintaining the integrity of the marketplace.
- Blockchain Network: The marketplace app interacts with the blockchain network on which the NFTs and associated smart contracts reside. It communicates with the network to execute transactions, transfer ownership, and retrieve information such as NFT details and transaction history.
- Off-chain Processing: The off-chain processing component handles operations that occur outside the blockchain, such as filling offers and placing bids. It manages the flow of information between the frontend, backend, and blockchain, ensuring the necessary data is processed and recorded accurately.
- Wallet Integration: Wallet integration enables users to connect their digital wallets to the marketplace app. This integration allows for secure storage of NFTs, signing transactions and data, and interacting with the blockchain.
Smart Contract Interaction
function buy(
Order memory order,
bytes memory sellerSignature,
address payableToken,
address signer,
uint256 gatedTokenId
) external payable nonReentrant
The buy
function enables users to purchase items listed in orders, validates the authenticity of the order, processes the payment, and fulfills the order by transferring the purchased item to the buyer.
Order memory order
: This parameter represents the details of the order to be purchased such as the item being sold, price, quantity, etc.bytes memory sellerSignature
: This parameter represents the signature of the seller for the order. It is used to verify the authenticity of the order and ensure that it was created and signed by the genuine seller.address payableToken
: This parameter represents the address of the token used for payment. It can be an ERC20 token contract address if the payment is made with a specific ERC20 token, or it can be the Ethereum (ETH) address if the payment is made with Ether.address signer
: This parameter represents the address of the signer or the entity who signed the order. It is used to validate the seller's signature.gatedTokenId
: This represents the gated TokenID
function sell(
Order memory order,
bytes memory buyerSignature,
uint256 expirationTime,
address receivableToken,
address paymentSettlementAddress
) external nonReentrant
- The
sell
function facilitates the acceptance of an offer for the given order by the seller. - It checks the validity of the provided
buyerSignature
by using the order details to verify the signature against the buyer's address. - The function then verifies if the offer is still valid by checking if the current block timestamp is before the specified
expirationTime
. - If the offer is valid and the signature is verified, the function proceeds to execute the payment process using the
receivableToken
. The seller receives the agreed-upon payment for accepting the offer. - `paymentSettlementAddress is represents the list of payment settlement address and its shares percentage.`
function executeAuction(
Order memory order,
bytes memory sellerSignature,
bytes memory buyerSignature,
address payableToken,
address sellerSigner,
BidHistory[] memory bidHistory
) external payable nonReentrant {
- The
executeAuction
function is responsible for handling the entire auction process, from verifying the authenticity of the auction order and the final bid to processing the auction settlement. - It first checks the validity of the provided
sellerSignature
by using thesellerSigner
address to verify the signature against the auction order details. - Next, it verifies the validity of the provided
buyerSignature
by using the auction order details to verify the signature against the buyer's address. This ensures that the final bid is genuine. - The function then proceeds to process the auction settlement, including transferring the highest bid amount to the seller as payment for the item and transferring the item to the winning bidder.
- The function may also update relevant state variables to reflect the auction's completion.
Getter Methods
GetRoyaltyInfo Function
function getRoyaltyInfo(address collectionAddress, uint256 tokenId)
external
view
returns (address payable[] memory recipients, uint256[] memory bps)
The getRoyaltyInfo
function retrieves the royalty information by calling the getRoyalty
function from the royaltySupport
contract (which is assumed to be of type IRoyaltyEngine
).
Note
This contract can be deployed using the above address. If you have any questions or require deployment assistance, please contact us at [email protected].
Updated 11 months ago