Onchain - Dutch Auction
Introduction
A Dutch auction is a unique type of auction where the price of an item starts high and gradually decreases until it reachs the reserved price. The Dutch auction was designed with the following goals,
- Fair Price Discovery
- Maximize Participation
- Buyer Flexibility
- Refunds for Overpayment
- Instant Delivery
- Multiple Currency Support
- Royalty Support
The Dutch Auction implentation consists of two components,
DutchAuction.Sol
This is the core contract of Auction Logic, which compresses the main functionalities of a Dutch auction, such as creating an auction, buying, ending, and extra features.
DutchUtility.sol.
This is the peripheral contract, which supports the validation and computation parts of the core contract.
Deployments
Ductch Auction contracts are deployed in Sepolia Testnets.
Details are,
Sepolia Testnet
Name of the Contract | Contract address |
---|---|
DutchAuction | 0xE19A6348AAB8914654E59Dbda3367A6b5dC0EA76 |
DutchUtility | 0x8679b108bF67fBA46231D154e4C7554320E757a7 |
Architecture Overview
The architecture of a Dutch auction typically consists of the following components:
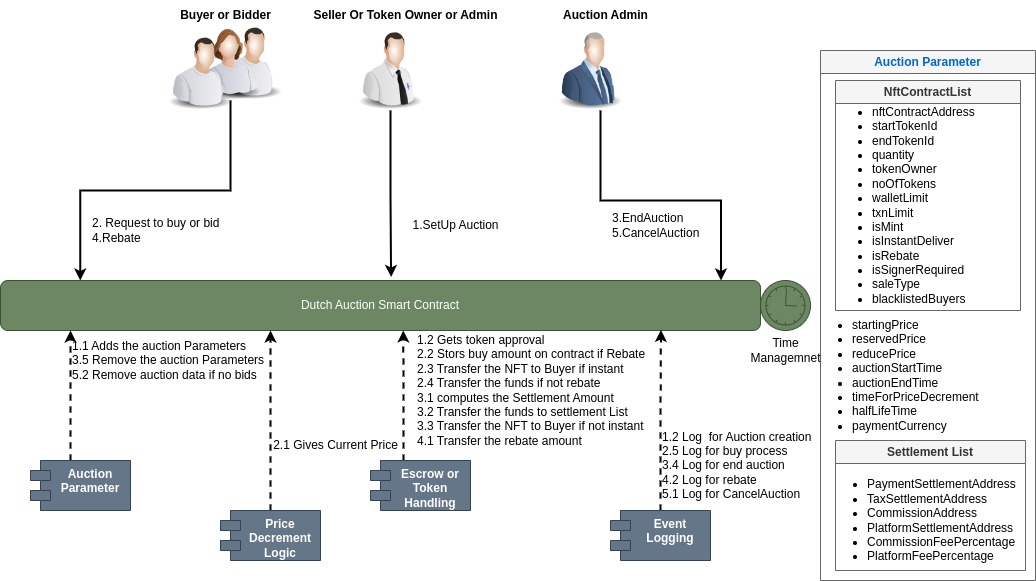
Auction Contracts: These are smart contracts that governs the auction process. It includes functions for starting and managing the auction, accepting bids, calculating the current price, and determining the winner.
Auction Parameters: The auction parameters are configurable variables that define the start price, end price, duration, and any other relevant parameters specific to the Dutch auction mechanism being implemented.
Bidders or buyer : Bidders or buyers are participants who submit bids during the auction. They interact with the auction contract through their Ethereum addresses and may need to sign their bids with their private keys to provide authenticity.
Price Decrement Logic: The auction contract includes a mechanism to decrement the price over time according to the specified rules of the Dutch auction. This logic could be linear, exponential, or follow any other predefined pattern.
Time Management: The auction contract utilizes the block timestamp to track the start time and end time of the auction. This allows the contract to determine the current phase of the auction and adjust the price accordingly.
Escrow or Token Handling: Depending on the auction's purpose, the contract may need to handle the escrow of funds or the transfer of tokens between the bidders and the auctioneer. This typically involves functions for accepting bids, releasing NFT to the buyer, releasing funds to token owners and refunding rebate amounts to bidders.
Event Logging: The auction contract may emit events to log important auction-related information, such as bid submissions, price changes, auction completion, and winner announcements. These events can be used for off-chain analysis and user notifications.
Smart Contract Interaction
The Dutch auction contracts facilitate the core functionalities of the Dutch auction. In terms of user aspects, the following methods are available:
Admin-related methods
function createOrUpdateDutchAuction(
string memory dutchAuctionID,
IDutchAuction.DutchAuctionList memory list
) external
The purpose of this function is to create a new Dutch auction or update an existing one within the smart contract. The dutchAuctionID
serves as a unique identifier for the auction, allowing the contract to keep track of multiple auctions if needed.The list
parameter is a structure or object containing the details of the Dutch auction.
Only callable by the admin of the Dutch auction contract or token owner(ie.Pre-Mint Type)
function endAuction(
string memory auctionId,
) external adminRequired
The endAuction
function is an external function in a smart contract designed for ending a Dutch auction. It takes a single parameter auctionId
, which is a string representing the identifier of the specific auction to be ended
function cancelAuction(
string memory auctionId
) external adminRequired;
The cancelAuction
function is designed to cancel a specific auction if there is no Sale or bids in auction concerned. It takes a single parameter auctionId
, which is a string representing the identifier of the auction to be canceled.
Buyer Related Methods
function buy(
string memory dutchAuctionID,
uint256 price,
uint256 tax,
uint256 quantity,
uint256 unit1155,
bytes32[] memory blacklistedProof,
IDutchAuction.Discount memory discount
) external payable
The purpose of buy
function is to allow a buyer to participate in a Dutch auction and purchase tokens. The dutchAuctionID
parameter is used to identify the specific Dutch auction in which the buyer wishes to participate. The price
represents the amount of funds or tokens the buyer wants to spend on the purchase.
The other parameters, such as tax
, quantity
, unit1155
, blacklistedProof
, and discount
, provide additional information related to the purchase, including any applicable fees, quantities, blacklisting proofs, and discounts.
To estimate the
price
before calling the buy function, the DutchUtility contracts provide support for determining the price using the functiongetCurrentDutchPrice(string memory dutchAuctionID).
function rebate(
string memory dutchAuctionID,
address buyer
) external
The purpose of rebate
function is to initiate a rebate or refund process for excess funds in a Dutch auction. When a buyer participates in a Dutch auction and pays more than the final price, the excess amount can be refunded back to the buyer's wallet address.
By calling this function and passing the dutchAuctionID
and buyer
, the rebate process is initiated for the specified auction and buyer address. The contract will verify the excess funds paid by the buyer and initiate the refund process by transferring the appropriate amount back to the specified wallet address.
The rebate function should be called after the auction ends by the admin, or when all listed tokens are sold out before the endTime.
Buyers can obtain the available refund amount by using the getter function
claimable
of the DutchUtility contract.
Getter Metthods
Getter functions are available in both the Dutch Auction and Dutch Utility contracts.
DutchAuction Methods
function getListings(
string memory dutchAuctionID
) external view returns (IDutchAuction.DutchAuctionList memory auctionist)
The purpose of the getListings
function is to retrieve the details of a specific Dutch auction listing. By passing the dutchAuctionID
, external callers can access the information related to that particular auction.
The function returns a DutchAuctionList
object that contains details such as auction duration, starting price, reserve price, and other relevant information specific to the Dutch auction.
function getLatestSaleInfo(
string memory dutchAuctionID
) external view returns (LatestSaleDetails memory saleDetails)
The function getLatestSaleInfo
retrieves the details of the latest sale for a Dutch auction identified by the given dutchAuctionID
. This includes the last sale price, number of NFTs sold, and other relevant information.
function getBuyerInfo(
string memory dutchAuctionID,
address BuyerAddress
) external view returns (BuyerDetails memory buyerDetails)
The function getBuyerInfo
retrieves the details of a specific buyer in a Dutch auction identified by the given dutchAuctionID
and BuyerAddress
When calling this function, the contract will return an object of type BuyerDetails
that contains the details of the buyer's participation, such as the bought price, token quality, tax paid, and discount-related information in the Dutch auction.
function getBuyerAddress(
string memory dutchAuctionID
) external view returns (address[] memory Buyers)
The getBuyerAddress
function retrieves the addresses of all buyers who participated in a specific Dutch auction identified by the dutchAuctionID
.
DutchUtility Methods
function getCurrentDutchPrice(
string memory dutchAuctionId
) public view returns (uint256 currentPrice)
External parties or smart contracts can retrieve the current price in a Dutch auction by utilizing getCurrentDutchPrice
. This allows them to monitor the auction progress, make informed decisions, or perform calculations based on the current price information.
function claimable(
string memory dutchAuctionID,
address buyer
) public view returns (uint256 totalClaimable, uint256 totalTax)
The claimable
function allows for calculating the total amount that can be claimed by a buyer and the total tax associated with it in a rebate-type auction.
Note
This contract can be deployed using the above address. If you have any questions or require deployment assistance, please contact us at [email protected].
Updated 7 months ago