SDK - Connect Wallet
How to Install and Setup the Mojito Connect-wallet SDK module.
Overview
The Connect-Wallet SDK is designed to simplify the process of integrating wallet connectivity into your application. It provides a unified interface that allows users to connect their wallets using various options, such as MetaMask, WalletConnect, and email-based connection.
This guide will help you better understand how to use and integrate the Connect-wallet SDK into your application.
Note:
For more details, you can refer to the NPM public repository here.
Prerequisite
Before proceeding with the installation steps, ensure that you have the following prerequisites in place:
- Node.js and NPM/Yarn: Make sure you have Node.js installed on your machine and and the Node.js version should be 18 or higher., along with either NPM (Node Package Manager) or Yarn. You can download and install Node.js from the official website here.
- API URL: Determine the appropriate API URL to use based on your environment.
If you are using create-react-app
Add below packages as dependencies in your package.json
{
...
"dependencies": {
...
"url": "latest",
"http": "npm:http-browserify",
"https": "npm:https-browserify",
"zlib": "npm:browserify-zlib",
"http-browserify": "latest",
"https-browserify": "latest",
"browserify-zlib": "latest",
"assert": "^2.0.0",
"stream": "^0.0.2"
}
}
To ignore the sourcemap warnings, create a .env file with the following in your root directory:
GENERATE_SOURCEMAP=false
Installation and Setup
Step 1: Install the Connect-wallet Module
Install the Mojito Connect-wallet SDK module on your project by using one of the following methods below:
Install using NPM:
npm i @mojito-inc/connect-wallet
Install using Yarn:
yarn add @mojito-inc/connect-wallet
Note:
Need to install following dependency for this module
yarn add @mui/[email protected] @mui/[email protected] @apollo/client graphql npm install @mui/[email protected] @mui/[email protected] @apollo/client graphql
Step 2: Wrap the Provider and Set API URL, Bearer Token and Theme
In your project's root file, follow these steps to wrap the provider and configure the API URL, Bearer token and Theme:
-
Determine the appropriate API URL based on your environment:
- For the development environment, set
API_URL
tohttps://api-dev.mojito.xyz/query
. - For the production environment, set
API_URL
tohttps://api.mojito.xyz/query
.
- For the development environment, set
-
Wrap the provider using the
ConnectWalletProvider
component from the Mojito Connect-wallet SDK.- Import the
ConnectWalletProvider
from@mojito-inc/connect-wallet
in your JavaScript file. - Use the
ConnectWalletProvider
component and provide the following props:uri
: Set this prop to the determinedAPI_URL
based on your environment.token
: Pass the obtained JWT token or Auth0 token as the value for this prop. Use the formatBearer <Token>
.
- Import the
import { ConnectWalletProvider } from "@mojito-inc/connect-wallet";
import { ThemeProvider } from '@mui/material/styles';
const [token, setToken] = useState<string>();
const client = useMemo(
() => ({
uri: Configuration.API_HOSTNAME,
token: token ? `Bearer ${ token }` : undefined,
}),
[token],
);
<ThemeProvider theme={ theme }>
<ConnectWalletProvider
projectId={ Configuration.PROJECT_ID } //wallet connect client id
clientOptions={ client }
onAuthenticated={ setToken }
theme={ theme } >
{ children }
</ConnectWalletProvider>
</ThemeProvider>
Step 3: Integrate Connect wallet
To use the Connect Wallet feature, follow these steps:
Import the necessary modules from the @mojito-inc/connect-wallet
package:
import { ConnectWalletContainer } from "@mojito-inc/connect-wallet";
<ConnectWalletContainer
open={ open }
config={ config }
walletOptions={ walletOptions }
image={ image }
isDisConnect={ isDisConnect }
walletAddress={ walletAddress }
onCloseModal={ onClickCloseModal } />
Hooks
Once wallet connected you can able to get wallet details from these hooks:
Network hooks
import { useNetwork } from '@mojitoinc/mojito-connect-wallet';
const { chainID, id, isTestnet, name } = useNetwork();
Wallet details hooks
import { useWallet } from '@mojitoinc/mojito-connect-wallet';
const { address, balance } = useWallet();
Provider hooks
import { useProvider } from '@mojitoinc/mojito-connect-wallet';
const { provider, providerType } = useProvider();
Note:
Once wallet connected , wallet details can get from hooks or can able to retrieve from session storage using key variable WalletDetails.
Fill in the required parameters for the ConnectWalletContainer
Parameter | Type | Required | Description |
---|---|---|---|
open | boolean | ✅ | to open modal |
config | Object | ✅ | Configuration |
walletOptions | Object | ✅ | walletOptions |
image | Object | ✅ | Image |
content | Object | to customise the contents in UI | |
isDisConnect | boolean | -✅ | to disconnect the wallet |
walletAddress | string | ✅ | connected wallet address |
isRefetchBalance | boolean | to re-fetch balance | |
onCloseModal | function | ✅ | function to handle close modal |
Fill in the required parameters for the config
Parameter | Type | Required | Description |
---|---|---|---|
orgId | string | ✅ | organisation id |
chainId | number | ✅ | chainId id for paper wallet |
paperClientId | string | ✅ | paper client id to connect with email |
paperNetworkName | Object | ✅ | paperNetworkName |
Fill in the required parameters for the paperNetworkName
Enum |
---|
Ethereum |
Sepolia |
Goerli |
Polygon |
Mumbai |
Fill in the required parameters for the walletOptions
Parameter | Type | Required | Description |
---|---|---|---|
enableMetamask | boolean | ✅ | to enable or disable metamask |
enableEmail | boolean | ✅ | to enable or disable email connect |
enableWalletConnect | boolean | to enable or disable wallet connect |
Fill in the required parameters for the Image
Parameter | Type | Required | Description |
---|---|---|---|
error | string | ✅ | error icon in svg, png, jpeg or gif format |
metamask | string | ✅ | metamask icon in svg, png, jpeg or gif format |
logo | string | ✅ | logo icon in svg, png, jpeg or gif format |
walletConnect | string | wallet connect logo icon in svg, png, jpeg or gif format |
Response
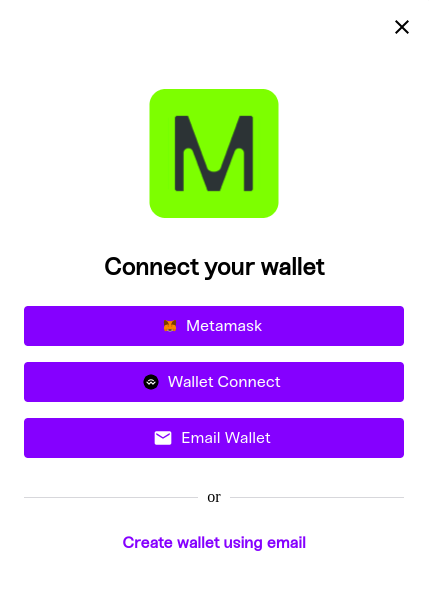
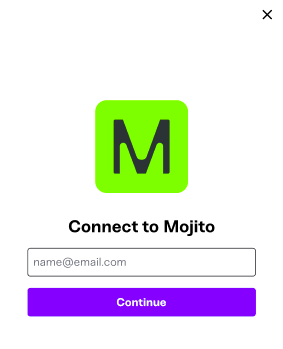
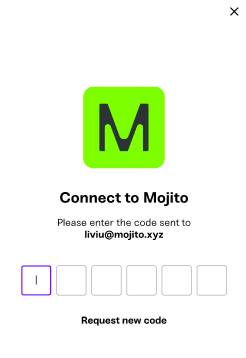
Note:
For complete documentation of the connect-wallet SDK offerings, see the connect-wallet.
Updated over 1 year ago