Onchain Buynow
Introduction
On-chain buy with a fixed price refers to a mechanism where buyers can purchase NFTs (Non-Fungible Tokens) directly from a smart contract on the blockchain at a predetermined fixed price. This approach eliminates the need for bidding or negotiating and allows for an instant purchase at the specified price.
Deployments
Sepolia Testnet
Name of the Contract | Contract address |
---|---|
OnchainBuy | 0x04BDeD25381E384aF4Ad6B88901CA1adb578A533 |
Architecture Overview
The architecture for an on-chainBuy typically involves the following components:
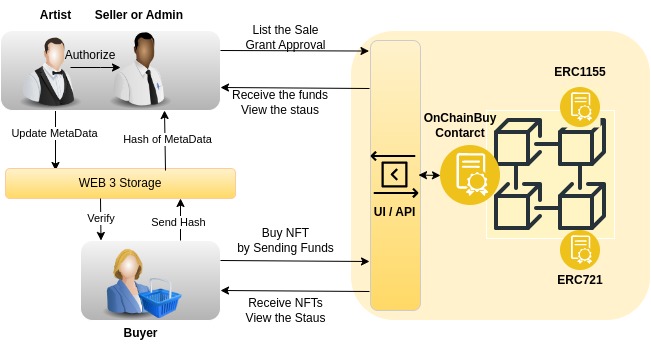
- OnchainBuy Contract: This is the smart contract deployed on the blockchain that implements the Buy functionality. It contains the necessary logic to handle the Buy process, including setting the fixed price, verifying payments, and facilitating the transfer of the NFT.
- NFT Contract: This represents the smart contract that manages the NFT, including its ownership and transfer functionalities. The OnchainBuy contract interacts with the NFT contract to facilitate the transfer of the NFT between the buyer and seller.
- Buyer: Represents the individual or entity interested in purchasing the NFT asset at the fixed price.
- Seller or Admin: Represents the individual or entity offering the NFT asset for sale.
- Blockchain Network: This is the underlying blockchain network, such as Ethereum, on which the BuyNow contract and NFT contract are deployed. It ensures the secure execution of transactions and maintains the immutability of the contracts' state.
Smart Contract Interaction
Admin Related Methods
function createOrUpdateSale(
PriceList calldata list,
string calldata saleId,
bool enableSignature
) external onlyOwnerorAdmin;
The createOrUpdateSale
function allows the contract owner or an admin to create a new sale or update an existing one. The PriceList
struct contains all the necessary details and preferences for the sale.
List
: This struct contains various attributes related to the sale, such as the range of NFT tokens, maximum cap, NFT contract address, minimum fiat price, minimum crypto price, payment currency options, settlement details, transaction status (mint or transfer), payment status (fiat or crypto) and token gating contract .
saleId
: Represents the unique identifier for the sale
enableSignature
: Indicates whether a signature is required for the sale or not.Setting this to true means that all transactions related to this sale require a signature for validation
function cancelSale(
uint256 saleId
) external;
The actual implementation of the cancelSale
function would involve finding the sale associated with the provided saleId
, performing any necessary checks (e.g., whether the sale exists, whether the caller has the right permissions to cancel the sale), and then executing the cancellation logic.
Buyer Related Methods
function buy(
BuyList memory list,
uint256 tax,
Discount calldata discount
) external
The buy
function is an external function in a smart contract that facilitates the purchase of an item or token. It takes three parameters: a BuyList
, a tax
value, and a Discount
BuyList
struct likely contains various details related to the purchase, such as the sale ID, token owner, token ID, token quantity, buyer's address, payment token and payment amount
tax
is representing the tax amount associated with the purchase.
The Discount
struct likely contains information about any discounts applied to the purchase, including the discount percentage, expiration time, nonce, signature, and the address of the signer (signer is the entity that issued the discount).
Getter Methods
function getListingPrice(string calldata saleId)
external
view
returns (
uint256[] memory minimumCryptoPrice,
address[] memory paymentCurrency
)
The purpose of getListingPrice
function is to provide a way for users to retrieve the listing price information for a specific sale based on the given saleId
. By calling this function, users can see the different payment methods and their corresponding minimum crypto prices for the sale
function getContractData()
external
view
returns (
address _platformAddress,
uint16 _platformFeePercentage,
IPriceFeed _priceFeedAddress,
IRoyaltyEngine _royaltySupport,
uint64 _max1155Quantity
)
The purpose of getContractData
function is to allow users or other contracts to easily query various contract-related data, such as the platform address, fee percentages, price feed contract, royalty engine contract, and any quantity restrictions for ERC-1155 tokens.
function getRoyaltyInfo(address collectionAddress, uint256 tokenId)
external
view
returns (address payable[] memory recipients, uint256[] memory bps)
{
(
recipients,
bps // Royalty amount denominated in basis points
) = royaltySupport.getRoyalty(collectionAddress, tokenId);
}
The getRoyaltyInfo
function retrieves the royalty information by calling the getRoyalty
function from the royaltySupport
contract (which is assumed to be of type IRoyaltyEngine
).
Note
This contract can be deployed using the above address. If you have any questions or require deployment assistance, please contact us at dev-support@mojito.xyz.
Updated 8 months ago