Onchain - Auction
Introduction
On-chain auctions have revolutionized the buying and selling of digital assets, especially non-fungible tokens (NFTs). Our motivation is to build and develop a blockchain-based auction using the decentralized Ethereum platform. The auction process is governed by a smart contract deployed on a blockchain platform like Ethereum. The smart contract defines the auction parameters, including the start time, end time, minimum bid, bid increments, and any additional rules specific to the auction. Bidders interact with the smart contract by submitting their bids directly from their wallets. Finally, the highest bidder wins the auction and gets the NFT from the token owner. The contract settles the funds to the settlement address concerned.
Feature
- Support ETH and ERC20 Support.
- Immutable Auction Rules.
- Bid Visibility.
- Automatic Bid Execution
- Automatic EndTime Extension
- Escrow and Payment Handling
Deployments
Sepolia Testnet
Name of the Contract | Contract address |
---|---|
OnchainAuction | 0xE39fD819A3a8D96f6dAcDE823CA1624Cd46a0486 |
Architecture Overview
The architecture for an on-chain auction typically involves the following components:
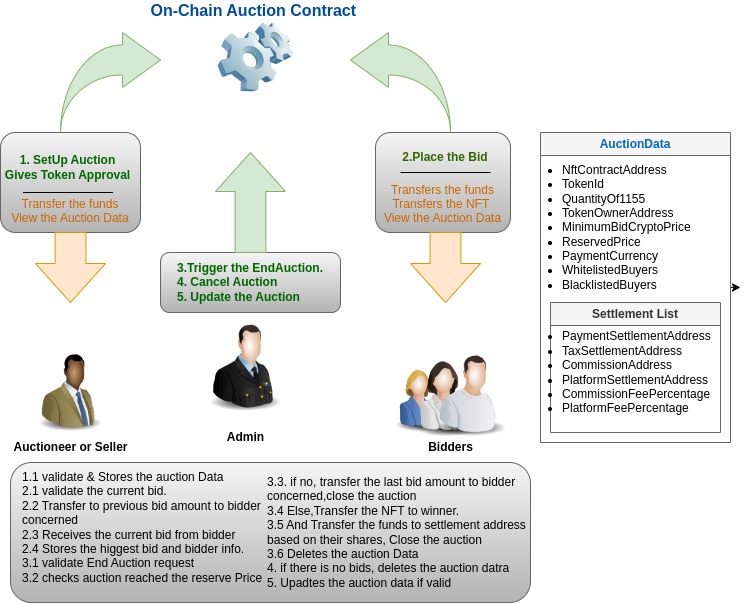
Auction Contract: This is the smart contract deployed on the blockchain that manages the auction process. It contains the necessary logic to handle bidding, determine the highest bidder, track the auction status, and distribute the assets after the auction ends.
Bidders: The participants who are interested in bidding for the auctioned assets. Each bidder interacts with the Auction Contract by submitting their bids.
Auction Manager or Admin : Represents authorized personnel or administrators with additional privileges in managing the auction system.
How it Works
The flow of the on-chain auction typically involves the following steps:
Auction Initialization: The Auction Manager or Admin initializes the auction by deploying the Auction Contract and specifying the auction parameters such as start time, end time, reserve price, or any other relevant parameters.
Bid Submission: Bidders interact with the Auction Contract by submitting their bids, either through direct function calls or via a user interface. Bids are typically accompanied by the bidder's address, bid amount, and any additional information required by the specific auction.
Bid Validation and Processing: The Auction Contract validates each bid, checking if it meets the minimum bid requirements, verifies the authenticity of the bidder if required, and determines if the bid is the highest bid at the moment. The contract updates the highest bid and highest bidder accordingly.
Auction Completion: When the auction end time is reached, either the Admin or the Highest Bidder must call EndAuction. The Auction Contract finalizes the auction by determining the winning bidder, transferring the assets to the winner, and handling any additional steps, such as distributing the proceeds from the auction or refunding unsuccessful bidders.
Throughout the process, the Auction Contract and other components interact with each other and the blockchain to ensure transparency, immutability, and security of the auction process.
Smart Contract Interaction
Admin Related Methods
function createAuction(
string calldata auctionId,
createAuctionList calldata list,
uint32 startTime,
uint32 endTime
) external nonReentrant {
The purpose of createAuction
function is to create a new auction with the specified parameters. The auctionId
serves as a unique identifier for the auction, allowing the contract to differentiate between multiple auctions if needed.
The list
parameter contains details and properties of the auction. These include the NFT or ERC-1155 token being auctioned, the token owner, minimum bid price, reserved price, payment currency, whitelist/blacklist for buyers, Auto Time Extension Parameter (durationForCheck & durationToIncrease) and the settlement mechanism.
The startTime
and endTime
parameters define the duration of the auction, with startTime
indicating the Unix timestamp when the auction begins and endTime
indicating the Unix timestamp when the auction ends.
function endAuction(string memory auctionId) external nonReentrant
The endAuction
function triggers the finalization process, which may include tasks such as determining the winning bidder, transferring the auctioned item or asset to the winning bidder, releasing any held funds or escrow, and updating the auction status to reflect that it has ended.
Buyer Related Methods
function bid(
string calldata auctionId,
uint256 bidAmount,
uint256 tax,
bytes32[] memory whitelistedProof,
bytes32[] memory blacklistedProof,
Approval calldata approval
) external payable nonReentrant
The bid
function allows a bidder to place a bid in an auction. Bidders provide the auction ID, bid amount, tax, and whitelistedProof & blacklistedProof (if applicable) and admin approval Parameters(expirationTime, nonce,signature & signer). The function is payable to send funds along with the bid.
Additional Auto Time Extension facility is added. if the user is processing the bid nearest to the end of Bid Time, It automatically increases the End time based TimeStamp set by auction level. It emits the event named endTimeExtended with old and new end Time
Getter Methods
The following public variables provide auction-related information to the users.
function bidderDetails(string memory auctionId);
This function returns information on the highest bidder and highest bid, as well as other bidder details.
function listings(string memory auctionId)
This function returns a list
as an object. The list
parameter contains details and properties of the auction. These include the NFT or ERC-1155 token being auctioned, the token owner, minimum bid price, reserved price, payment currency, whitelist/blacklist for buyers, and the settlement mechanism.
Note
This contract can be deployed using the above address. If you have any questions or require deployment assistance, please contact us at [email protected].
Updated 6 months ago