Claim Management Kit
⚙️ How to Install and Setup the Mojito Claim-management SDK module.
Overview
The Mojito Claim Management SDK offers abstractions that facilitate interactions with claim tokens in a TypeScript/JavaScript environment. This SDK primarily focuses on handling claim tokens. It also provides various options for connecting to a wallet, such as an email connection, crossmint, Metamask, Email wallet, and Wallet Connect.
This guide will help you better understand how to use and integrate the Claim-management SDK into your application.
Prerequisite
Before proceeding with the installation steps, ensure that you have the following prerequisites in place:
- Node.js and NPM/Yarn: Make sure you have Node.js installed on your machine, and the Node.js version should be 18 or higher, along with either NPM (Node Package Manager) or Yarn. You can download and install Node.js from the official website here.
- Authentication Provider: To obtain the necessary token for authentication, you need to have an authentication provider set up. This can be a JWT (JSON Web Token) or an Auth0 token, which will be passed as the "token" property in the configuration.
- API URL: Determine the appropriate API URL to use based on your environment.
- NEXT js: We are recommended to use Next.js for this SDK.
- Wallet: Connecting with the Mojito Claim Management SDK requires a wallet. Choose one of the following wallet options:
- MetaMask
- Wallet Connect
- Email Wallet
- Crossmint
Installation and Setup
Step 1: Install the Claim-management Module
Install the Mojito Claim-management SDK module on your project by using one of the following methods below:
Install using NPM:
npm install @mojito-inc/claim-management
Install using Yarn:
yarn add @mojito-inc/claim-management
Step 2: Connect Wallet
- After installing, connect your wallet by clicking the Connect your existing wallet button.
- Select the wallet that you want to connect to Mojito.
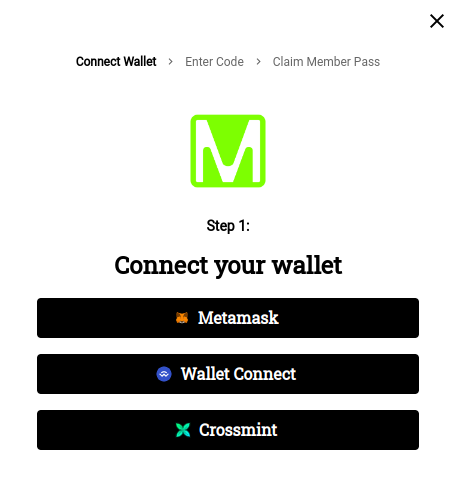
- Read and Agree to the terms and click on the claim now to claim an item.
- After claimed you will get an success modal.
Step 3: Wrap the Provider in the Root File
To integrate the Mojito Claim Management SDK into your application, follow these steps:
-
In the root file of your application, wrap the provider component. This step allows you to connect to the claim management APIs.
-
Choose the appropriate API URL based on your environment:
- Sandbox Environment: Use the API URL
https://api-sandbox.mojito.xyz/query
. - Production Environment: Use the API URL
https://api.mojito.xyz/query
.
- Sandbox Environment: Use the API URL
-
Obtain a JWT token or Auth0 token from your authentication provider. This token will be used for authorization purposes.
-
Wrap the provider using the
ClaimManagementProvider
component from the Mojito Claim Management SDK.
Basic setup example:
import { ClaimManagementProvider } from "@mojito-inc/claim-management";
import { useState, useMemo } from "react";
function App() {
const token = useState();
const client = useMemo(function() {
return {
uri: "API_URL", // API url
token: token || undefined // bearer token
};
}, [token]);
// Note: you can get the bearer token from local storage with a key "token"
}
Provider configuration:
// ClaimManagementProvider component usage
const providerProps = {
theme: theme, // To customise the theme refer below document
clientOptions: client,
onAuthenticated: setToken,
activeChain: activeChain,
clientId: clientId,
walletConnectProjectId: walletConnectProjectId // project id from wallet connect
};
Step 4: Using the ClaimTokenModal Component
To utilize the ClaimTokenModal component from the Mojito Claim Management SDK, follow these steps:
- Import the
ClaimTokenModal
component from the@mojito-inc/claim-management
module.
import { ClaimTokenModal } from "@mojito-inc/claim-management";
- Pass the required parameters to the
ClaimTokenModal
component.
Basic configuration:
const claimModalConfig = {
open: true,
name: "tokenName",
userEmail: "[email protected]",
config: configuration,
onCloseModal: handleCloseModal,
onSuccess: handleSuccess,
onClickBuyToken: handleBuyToken,
isDisConnect: false,
walletOptions: walletOptions,
link: linkConfig,
claimCode: "CLAIM123",
content: contentConfig,
claimType: "customcode"
};
React Hooks
useWallet hooks
To get the connected wallet details:
import { useWallet } from "@mojito-inc/claim-management";
const walletData = useWallet();
// walletData contains: address, balance, networkDetails, provider, providerType
useTransaction hooks
To get the transaction details:
import { useTransaction } from "@mojito-inc/claim-management";
const transactionData = useTransaction();
// transactionData contains: error, fetchInvoiceDetail, transactionDetails
Parameters
Params | Type | Required | Description |
---|---|---|---|
open | boolean | ✅ | to open or close the modal |
config | object | ✅ | Configuration |
onCloseModal | event() | ✅ | to close the modal |
isDisConnect | boolean | ✅ | pass true to disconnect wallet |
walletOptions | object | ✅ | wallet Options to enable or disable wallet options |
link | object | ✅ | link: redirection in app |
claimCode | string | pass claim code here if saleType is customcode | |
listingId | string | pass the listing id if claim with no code | |
claimType | enum | pass the claim type | |
isClaimWithGas | boolean | claim free token | |
content | object | content: to customise the content inside the modal | |
firstName | string | user first name | |
lastName | string | user last name | |
userEmail | string | user email | |
loginWithPersonalInformation | boolean | pass true if wants to login with personal information | |
tokenGatingConfig | object | create gating and pass the group and rule id | |
onSuccess | function | to handle own logic for the success modal button |
Configuration
Params | Type | Required |
---|---|---|
chainId | number | ✅ |
orgId | string | ✅ |
crossmintApiKey | string | |
crossmintEnv | string | |
paperClientId | string | |
paperNetworkName | Enum |
Wallet Options
Params | Type | Required |
---|---|---|
enableMetamask | boolean | ✅ |
enableWalletConnect | boolean | ✅ |
enableCrossmint | boolean | ✅ |
enablePaper | boolean | ✅ |
Link
Params | Type | Required |
---|---|---|
viewTokenTrackerURL | string | ✅ |
termsUrl | string | ✅ |
logoUrl | string | ✅ |
Content
Params | Type | Required | Description |
---|---|---|---|
ClaimCodeContent | object | contentData: to customise the title and description of claim modal | |
ConnectWalletContent | object | contentData: to customise the title and description of wallet modal | |
SuccessContent | object | contentData: to customise the title and description of success modal | |
LoaderContent | object | contentData: to customise the title and description of loader modal | |
RecoveryCodeModal | object | contentData: to customise the description of recovery modal | |
TokenGatingContent | object | contentData: to customise the description of token gating eligible modal | |
TokenGatingNotEligibleContent | object | contentData: to customise the description of token gating not eligible modal | |
ClaimWithGasContent | object | contentData: to customise the description of claim with gas modal | |
ClaimErrorContent | object | contentData: to customise the description of claim error modal |
Content Data
Params | Type | Required |
---|---|---|
title | string | |
description | string |
Theme Config
Basic theme configuration:
import { createTheme } from '@mui/material/styles';
const theme = createTheme({
typography: {
fontFamily: 'Slate',
},
palette: {
primary: {
main: '#FDCC35',
},
secondary: {
main: '#356045',
},
background: {
default: '#000',
},
text: {
primary: '#000',
}
}
});
Complete theme configuration:
For a complete theme configuration with all MUI component overrides, please refer to the full documentation or the example provided in the NPM package.
TypeScript Support:
Note: If you are faced any type issue in theme, create a file called "mui.d.ts" in root or theme folder and paste the below code:
import '@mui/material/styles';
interface MojitoClaimManagementTheme {
delivery: {
textColor: string;
tokenTrackerColor: string;
};
Discount?: {
inProgressColor: string;
inProgressBackground: string;
};
error?: string;
deliveryBackgroundColor?: string;
linkColor?: string;
success: string;
font: {
primary: string;
secondary: string;
tertiary: string;
};
}
declare module '@mui/material/styles' {
export interface Theme {
MojitoClaim?: MojitoClaimManagementTheme
}
export interface ThemeOptions {
MojitoClaim?: MojitoClaimManagementTheme
}
}
Note:For complete documentation of the SDK offerings, see the claim-management.
Updated 23 days ago